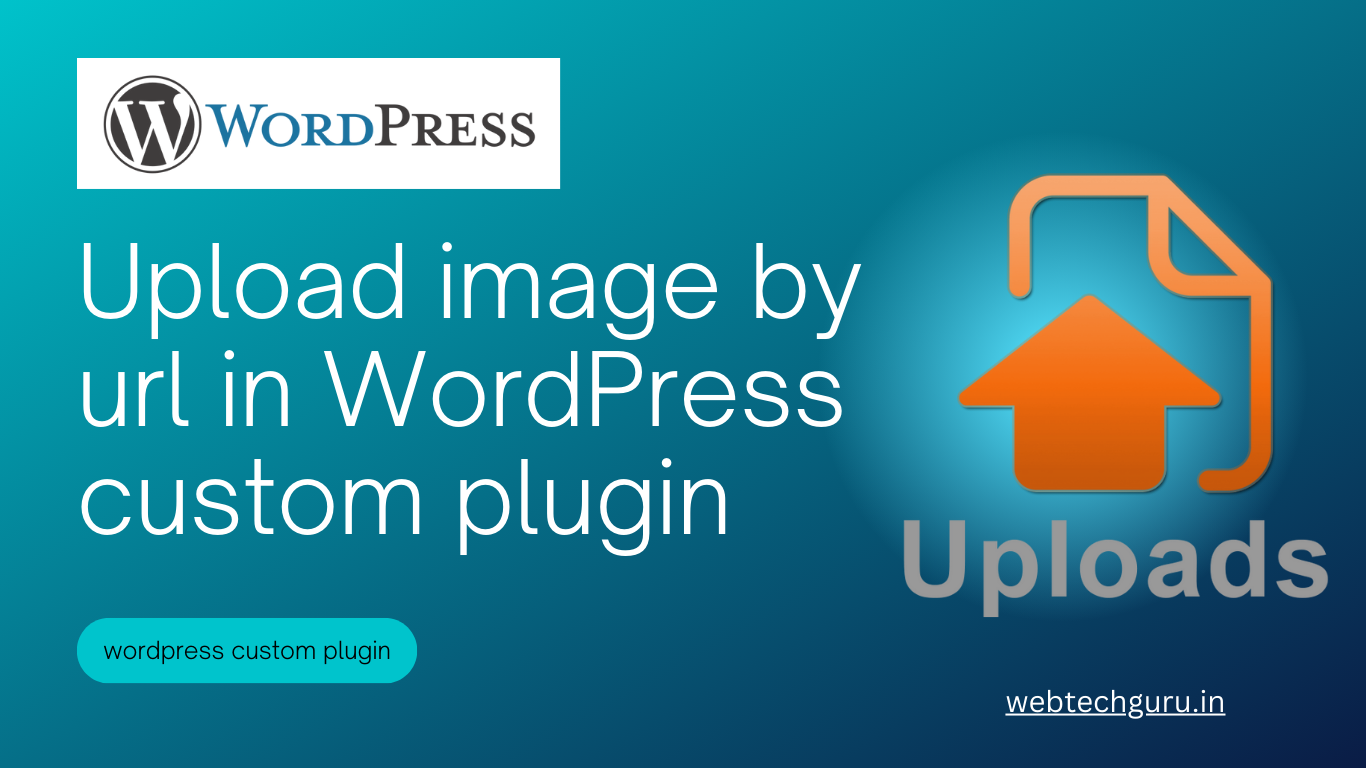
How to upload image by url in WordPress custom plugin
Certainly! Creating a custom plugin in WordPress to upload an image by URL involves several steps. Below is a step-by-step guide to achieve this:
Step 1: Set up Your Plugin
Create a new directory in the `wp-content/plugins/` directory of your WordPress installation. Inside this directory, create a new PHP file for your plugin. For example, `custom-plugin.php`.
Step 2: Define Plugin Header
In your PHP file, start by defining the plugin information in the header. This includes the plugin name, description, version, etc.
<?php /* Plugin Name: Custom Plugin Description: A simple custom WordPress plugin. Version: 1.0 Author: Your Name */ ?>
Step 3: Create an Admin Page
Create an admin page to allow users to input the image URL and trigger the upload process.
register_activation_hook(__FILE__, 'custom_plugin_activate'); register_deactivation_hook(__FILE__, 'custom_plugin_deactivate'); function custom_plugin_activate() { // Activation code goes here } function custom_plugin_deactivate() { // Deactivation code goes here } add_action('admin_menu', 'custom_plugin_menu'); function custom_plugin_menu() { add_menu_page( 'Custom Plugin Settings', 'Custom Plugin', 'manage_options', 'custom-plugin-settings', 'custom_plugin_settings_page' ); }
Step 4: Create a Function to Handle Image Upload
Define a function that will handle the image upload by URL.
function custom_plugin_settings_page() { // Settings page content goes here echo '<div class="wrap"><h2>Custom Plugin Settings</h2><p>This is the settings page content.</p>'; ?> <p id="err-msg" style="color:#eb3233;font-weight: bold;"></p> <p id="succ-msg" style="color:green;"></p> <form id="img-upload-form"> <input type="url" name="img-url" id="img-url"> <input type="submit" id="img-upload-btn" value="Upload Image" class="button-primary"> </form> <script> jQuery(document).ready(function($){ $('#img-upload-btn').click(function(e){ e.preventDefault(); img_url = $('#img-url').val(); $('#succ-msg').html(''); // CHECK IF IMAGE URL VALE IS EMPTY if(img_url == ''){ $('#err-msg').text('Image Url is Required.'); }else{ $.ajax({ url:'<?php echo admin_url('admin-ajax.php'); ?>', type:'post', data:{action:'upload_img',data:img_url}, success:function(response){ $('#img-upload-form').trigger('reset'); if(response.success == false){ $('#err-msg').text(response.data); }else{ $('#succ-msg').html('<strong>'+response.message+'<br>Your Image Url is : </strong>'+ response.data); //console.log(response); } } }) } }); $('#img-url').keyup(function(){ $('#err-msg').text(''); $('#succ-msg').text(''); }) }) </script> <?php echo '</div>'; } // START CODE FOR UPLOAD IMAGE BY AJAX add_action('wp_ajax_upload_img', 'upload_img_by_ajax'); add_action('wp_ajax_nopriv_upload_img', 'upload_img_by_ajax'); function upload_img_by_ajax() { if (isset($_POST['data'])) { $img_url = esc_url_raw($_POST['data']); // Use esc_url_raw() for sanitizing the URL // GET FILE CONTENT $image_content = file_get_contents($img_url); if ($image_content !== false) { $random_number = rand(0, 99999); // Create a unique file name for the image based on $ticke_logo_name $file_name = $random_number . ".jpg"; // Upload the image to the WordPress media library $upload_dir = wp_upload_dir(); $upload_path = $upload_dir['path'] . '/' . $file_name; $uploaded = file_put_contents($upload_path, $image_content); if ($uploaded !== false) { $upload_url = $upload_dir['url'] . '/' . $file_name; $data = array('success'=>true,'data'=>$upload_url,'message'=>'Image Uploaded successfuly.'); //$data = json_encode($data); wp_send_json($data); } else { wp_send_json_error('Error uploading image.'); } } else { wp_send_json_error('Please check your image path.'); } } wp_die(); }
Step 5: Activate the Plugin
Activate your plugin through the WordPress admin panel.
Now, when you go to the “Custom Plugin” menu in the admin, you’ll find a form to input the image URL. Upon submission, the plugin will attempt to upload the image, and the result will be displayed.
Note: This is a basic example, and you may want to add more features like error handling, validation, and security checks based on your specific requirements.
Full Code
<?php /* Plugin Name: Custom Plugin Description: A simple custom WordPress plugin. Version: 1.0 Author: Your Name */ register_activation_hook(__FILE__, 'custom_plugin_activate'); register_deactivation_hook(__FILE__, 'custom_plugin_deactivate'); function custom_plugin_activate() { // Activation code goes here } function custom_plugin_deactivate() { // Deactivation code goes here } add_action('admin_menu', 'custom_plugin_menu'); function custom_plugin_menu() { add_menu_page( 'Custom Plugin Settings', 'Custom Plugin', 'manage_options', 'custom-plugin-settings', 'custom_plugin_settings_page' ); } function custom_plugin_settings_page() { // Settings page content goes here echo '<div class="wrap"><h2>Custom Plugin Settings</h2><p>This is the settings page content.</p>'; ?> <p id="err-msg" style="color:#eb3233;font-weight: bold;"></p> <p id="succ-msg" style="color:green;"></p> <form id="img-upload-form"> <input type="url" name="img-url" id="img-url"> <input type="submit" id="img-upload-btn" value="Upload Image" class="button-primary"> </form> <script> jQuery(document).ready(function($){ $('#img-upload-btn').click(function(e){ e.preventDefault(); img_url = $('#img-url').val(); $('#succ-msg').html(''); // CHECK IF IMAGE URL VALE IS EMPTY if(img_url == ''){ $('#err-msg').text('Image Url is Required.'); }else{ $.ajax({ url:'<?php echo admin_url('admin-ajax.php'); ?>', type:'post', data:{action:'upload_img',data:img_url}, success:function(response){ $('#img-upload-form').trigger('reset'); if(response.success == false){ $('#err-msg').text(response.data); }else{ $('#succ-msg').html('<strong>'+response.message+'<br>Your Image Url is : </strong>'+ response.data); //console.log(response); } } }) } }); $('#img-url').keyup(function(){ $('#err-msg').text(''); $('#succ-msg').text(''); }) }) </script> <?php echo '</div>'; } // START CODE FOR UPLOAD IMAGE BY AJAX add_action('wp_ajax_upload_img', 'upload_img_by_ajax'); add_action('wp_ajax_nopriv_upload_img', 'upload_img_by_ajax'); function upload_img_by_ajax() { if (isset($_POST['data'])) { $img_url = esc_url_raw($_POST['data']); // Use esc_url_raw() for sanitizing the URL // GET FILE CONTENT $image_content = file_get_contents($img_url); if ($image_content !== false) { $random_number = rand(0, 99999); // Create a unique file name for the image based on $ticke_logo_name $file_name = $random_number . ".jpg"; // Upload the image to the WordPress media library $upload_dir = wp_upload_dir(); $upload_path = $upload_dir['path'] . '/' . $file_name; $uploaded = file_put_contents($upload_path, $image_content); if ($uploaded !== false) { $upload_url = $upload_dir['url'] . '/' . $file_name; $data = array('success'=>true,'data'=>$upload_url,'message'=>'Image Uploaded successfuly.'); //$data = json_encode($data); wp_send_json($data); } else { wp_send_json_error('Error uploading image.'); } } else { wp_send_json_error('Please check your image path.'); } } wp_die(); }